Java Micronaut REST Server with PostgreSQL
đź•“ 45 minutes
What you’ll learn​
How to set up your application for :
- connecting to PostgreSQL database,
- getting data from REST API,
- providing data to REST API.
In this tutorial, we will create a simple java component with Java Micronaut Data scaffolder with connection to PostgreSQL database storage. We want to expose a single REST endpoint for getting the basic client data information, creating a microservice CRUD layer above DB storage.
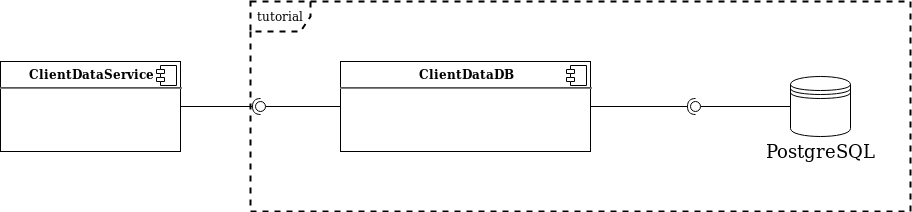
Project source​
This example project can be cloned from: http://gitlab.cloud.codenow.com/public-docs/client-authorization-demo/client-data-db.git
Prerequisites​
- Prepare your local development environment for CodeNOW with Micronaut.
- Follow the tutorial instructions in the Java Micronaut Local Development tutorial.
- Run PostgreSQL locally.
- You can run PostgreSQL directly or using docker compose.
- Configuration file for docker-compose can be downloaded from the link that can be found in the section Docker compose and third-party tools of the Java Micronaut Local Development tutorial.
- Create a new component
- For details see the section Prerequisites of the Java Micronaut Local Development tutorial.
Steps​
Open your IDE, import created component and start coding:
-
Define jpa entity
Client
. This simple table will store basic client data:-
Generate getters and setters with your IDE
package io.codenow.client.data.db.service.repository.entity;
import java.time.LocalDate;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
@Entity
public class Client {
@Id
@GeneratedValue
private Long id;
private String username;
private String firstname;
private String surname;
private LocalDate birthdate;
}
-
-
Create a new
ClientRepository
, which is a basic CRUD interface for micronaut data DB access:package io.codenow.client.data.db.service.repository;
import io.codenow.client.data.db.service.repository.entity.Client;
import io.micronaut.data.jdbc.annotation.JdbcRepository;
import io.micronaut.data.repository.CrudRepository;
import io.reactivex.Maybe;
@JdbcRepository
public interface ClientRepository extends CrudRepository<Client, Long> {
Maybe<Client> findByUsername(String username);
} -
Create a new controller and put all the parts together
-
For more details about Micronaut controller, see: https://docs.micronaut.io/latest/guide/index.html#httpServer
package io.codenow.client.data.db.service.controller;
import javax.inject.Inject;
import javax.validation.constraints.NotNull;
import io.codenow.client.data.db.service.repository.ClientRepository;
import io.codenow.client.data.db.service.repository.entity.Client;
import io.micronaut.http.annotation.Consumes;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
import io.micronaut.http.annotation.PathVariable;
import io.micronaut.http.annotation.Produces;
import io.micronaut.validation.Validated;
import io.reactivex.Flowable;
import io.reactivex.Maybe;
/**
* ClientDataController.
*/
@Validated
@Controller("/db")
@Produces
@Consumes
public class ClientDataController {
private final ClientRepository clientRepository;
@Inject
public ClientDataController(ClientRepository bookRepository) {
this.clientRepository = bookRepository;
}
@Get("/clients")
public Flowable<Client> listClients() {
return Flowable.fromIterable(clientRepository.findAll());
}
@Get("/clients/{username}")
public Maybe<Client> getClient(@PathVariable @NotNull String username) {
return clientRepository.findByUsername(username);
}
}
-
-
Next prepare database configuration:
-
Go to the PgAdmin console (http://localhost:5050 if using compose-postgre from our Local development manual) and create a new db client-db with the scheme
client-data
. -
Add maven dependency to your
pom.xml
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.11</version>
</dependency>
-
-
Now change the local configuration in
codenow/config/application.yaml
:-
Fill
{db user}
and{db password}
according to your local development setupdangerThese settings are valid only in your local development environment to connect to your local database! Never store your credentials in the codebase! You don't have to implement your own solution for the secure storage of credentials. In CodeNOW, credentials are securely stored for each deployed service (database/message broker/...). You can find how to set up the service connection in CodeNOW in the Deployment Tutorial and in the Connecting Services Tutorial.
-
Make sure you follow yaml syntax (especially whitespaces)
datasources:
default:
url: jdbc:postgresql://localhost:5432/client-db?currentSchema=client-data
driverClassName: org.postgresql.Driver
username: {db user}
password: {db password}
schema-generate: CREATE
dialect: POSTGRES
-
-
Do not forget to change the
swagger.yaml
. Check it in the example project:src/main/resources/META-INF/swagger/swagger.yaml
-
Try to build and run the application in your IDE. After startup, you should be able to access your new controller’s swagger: http://localhost:8080/swagger/index.html
- For correct setup, check
README.md
in project root or see the tutorial Java Micronaut Local Development
- For correct setup, check
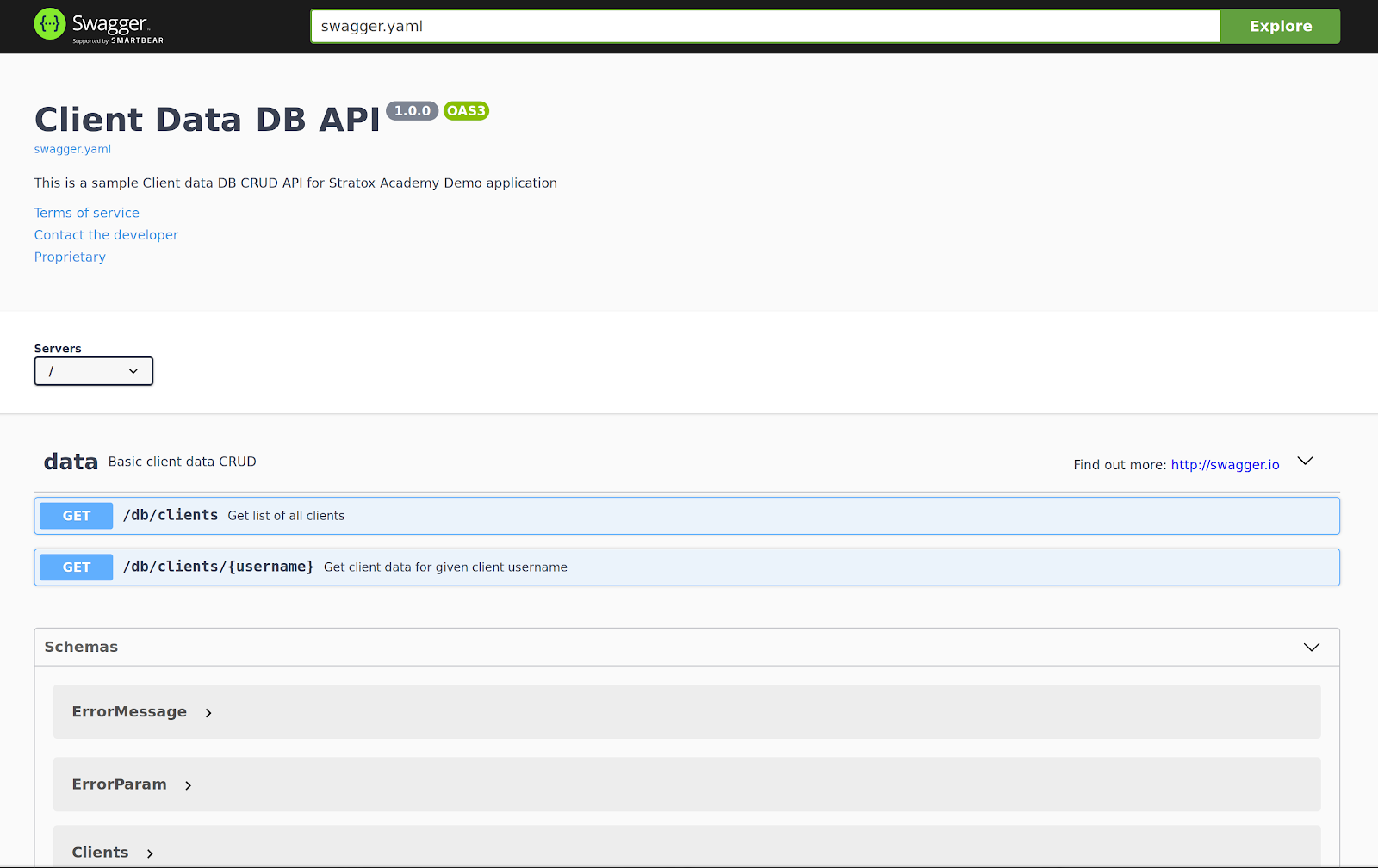
Deploy to CodeNOW​
If your code works in the local development, you are ready to push your changes to GIT and try to build and deploy your new component version to the CodeNOW environment.
- Check the Get New PostgreSQL user manual to get CodeNOW managed component properties.
- Make sure to change the application.yaml properties from the local to the production setup.
- For more information about application deployment see Application Deployment and Deployment Monitoring tutorials.
What's next?​
See the tutorial Micronaut REST server with Redis and Kafka.