Java Spring Boot REST API
đź•“ 45 minutes
What you’ll learn​
How to set up your application for:
- getting data from REST API,
- providing data to REST API.
In this tutorial, we will create a simple Java component with the Java Spring Boot scaffolder. We want to expose the single REST endpoint for getting user details for his username only. This will require simple sequential orchestration of two REST services, one to get user roles and the second for basic user details.
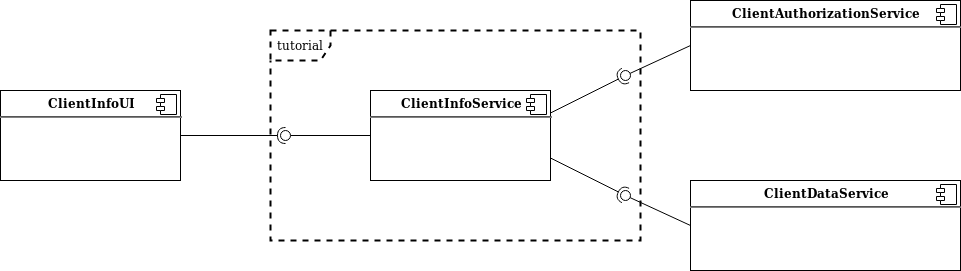
Project source​
This example project can be cloned from: http://gitlab.cloud.codenow.com/public-docs/java-spring-boot-demo/java-spring-boot-rest-api.git
Prerequisites​
- Prepare your local development environment for CodeNOW with Spring Boot.
- Follow the tutorial instructions in the Java Spring Boot Local Development tutorial.
- Create a new component.
- For details see the section Prerequisites of the Java Spring Boot Local Development tutorial.
Steps​
Open your IDE, import the created component and start coding:
-
Define the message payload. Here is an example of
ClientInfo
, which is a simple POJO with basic user details and roles:-
Generate getters and setters with your IDE.
-
Examples of the
ClientData
andClientAuthorization
classes can be found in the example project repository.package org.example.service.model;
import java.time.LocalDate;
import java.util.Set;
public class ClientInfo {
private String username;
private String firstname;
private String surname;
private LocalDate birthdate;
private Set<String> roles;
}
-
-
Next, create the classes
ClientDataService
andClientAuthorizationService
with the@Service
annotation. These classes will represent http clients for calling the orchestrated endpoints. Example forClientDataService
:-
For more details about Spring Boot
@Service
annotation, visit this page: https://docs.spring.io/spring/docs/current/javadoc-api/org/springframework/stereotype/Service.html -
For more details about Spring Boot WebClients use this link: https://docs.spring.io/spring-boot/docs/2.1.0.RELEASE/reference/html/boot-features-webclient.html
-
Create a new Java class:
package org.example.service.service;
import org.example.service.model.ClientData;
import org.springframework.stereotype.Service;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Flux;
@Service
public class ClientDataService {
private final WebClient webClient;
public ClientDataService(WebClient.Builder webClientBuilder) {
this.webClient = webClientBuilder.baseUrl("http://client-data-service").build();
}
public Flux<ClientData> getClientData(String username) {
return this.webClient
.get()
.uri("/data/{username}", username)
.retrieve()
.bodyToFlux(ClientData.class);
}
}
-
-
In the
baseUrl()
, you should put the url to your existing component. -
Create a new controller and put all parts together:
-
For more details about Spring Boot controllers, see: https://docs.spring.io/spring/docs/3.0.0.M4/reference/html/ch15s03.html
-
Create a new class
ClientInfoController
:package org.example.service.controller;
import org.example.service.model.ClientData;
import org.example.service.model.ClientInfo;
import org.example.service.model.ClientAuthorization;
import org.example.service.service.ClientDataService;
import org.example.service.service.ClientAuthorizationService;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import reactor.core.publisher.Flux;
@RestController
@RequestMapping("data")
public class ClientInfoController {
private Logger log = LoggerFactory.getLogger(ClientInfoController.class);
private ClientDataService clientDataService;
private ClientAuthorizationService clientAuthorizationService;
public ClientInfoController(ClientDataService clientDataService, ClientAuthorizationService clientAuthorizationService) {
this.clientDataService = clientDataService;
this.clientAuthorizationService = clientAuthorizationService;
}
@GetMapping("/clients/{username}")
public Flux<ClientInfo> getClientInfo(@PathVariable String username) {
log.info("GET client by username: {}", username);
Flux<ClientData> clientDataFlux = clientDataService.getClientData(username);
Flux<ClientAuthorization> clientRolesFlux = clientAuthorizationService.getClientAuthorization(username);
return clientDataFlux.zipWith(clientRolesFlux, (data, roles) -> {
ClientInfo response = new ClientInfo();
response.setUsername(data.getUsername());
response.setFirstname(data.getFirstname());
response.setSurname(data.getSurname());
response.setBirthdate(data.getBirthdate());
response.setRoles(roles.getRoles());
return response;
});
}
}
-
-
Try to build and run the application in your IDE. After startup, you should be able to access your new controller's swagger: http://localhost:8080/swagger/index.html
- For the correct setup, check the
README.md
file in the project root or see the tutorial Java Spring Boot Local Development, section Prepare local development IDE. - The component exposes a simple GET endpoint and can be tested using a browser call only. You should not forget to document your API and use the swagger-ui accordingly.
- For the correct setup, check the
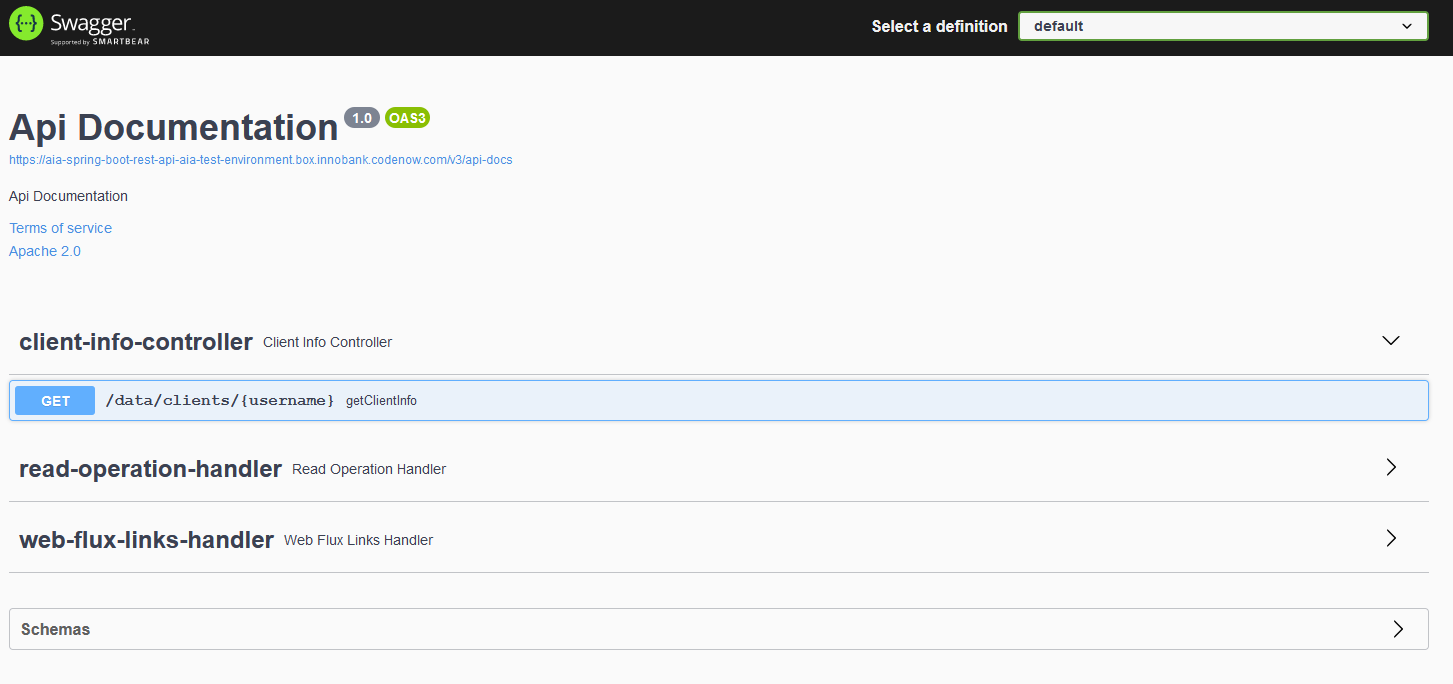
Deploy to CodeNOW​
If your code works in the local development, you are ready to push your changes to GIT and try to build and deploy your new component version to the CodeNOW environment.
- For more information about application deployment, see the Application Deployment and Deployment Monitoring tutorials.
What’s next?​
See our other developer guides: