Java Spring Boot REST Server with Redis
đź•“ 40 minutes
What you’ll learn​
How to set up your application for:
- connecting to Redis,
- getting data from REST API,
- providing data to REST API.
In this tutorial, we will create a simple java component with the Java Spring Boot scaffolder. We want to expose two REST endpoints for creating and getting user details. As we will store the user data in the Redis key-value storage, we need a client configuration for our component. The image below shows the diagram of the future application.
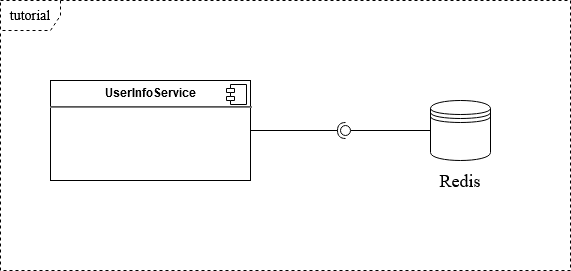
Project source​
This example project can be cloned from: http://gitlab.cloud.codenow.com/public-docs/java-spring-boot-demo/java-spring-boot-rest-server-with-redis.git
Prerequisites​
- Prepare your local development environment for CodeNOW with Spring Boot.
- Follow the instructions in the Java Spring Boot Local Development tutorial.
- Run Redis locally.
- You can run Redis directly or using docker compose.
- The configuration file for docker-compose can be downloaded from the link that can be found in the section Docker compose and third-party tools of the Java Spring Boot Local Development tutorial.
- Create a new component
- For details, see the section Prerequisites of the Java Spring Boot Local Development tutorial.
Steps​
-
Open your IDE, import the created component and start coding:
-
Define the entity
User
. This simple entity will have two values: username and a list of roles.- Generate getters and setters with your IDE.
import java.util.List;
public class User {
private String username;
private List<String> roles;
// getters + setters ...
}
-
-
Next prepare the configuration for the Redis client:
-
For more details about Spring Boot Redis, see: https://spring.io/projects/spring-data-redis
-
Add maven dependency to your
pom.xml
.- This dependency is the starter for using the Redis key-value data store with Spring Data Redis and the Lettuce client:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
<version>2.3.3.RELEASE</version>
</dependency> -
Create a new configuration class for redis
RedisConfig.class
:
import io.lettuce.core.RedisClient;
import io.lettuce.core.RedisURI;
import io.lettuce.core.api.StatefulRedisConnection;
import io.lettuce.core.api.sync.RedisCommands;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.repository.configuration.EnableRedisRepositories;
@Configuration
@EnableRedisRepositories
public class RedisConfig {
@Value("${spring.redis.url}")
private String uri;
@Bean
public RedisCommands connectionFactory() {
RedisURI redisURI = RedisURI.create(uri);
RedisClient redisClient = RedisClient.create(redisURI);
StatefulRedisConnection<String, String> redisConnection = redisClient.connect();
return redisConnection.sync();
}
} -
-
Create a new controller and put all the parts together
- For more details about the Spring Boot controller, see: https://spring.io/guides/gs/rest-service/
import io.lettuce.core.api.sync.RedisCommands;
import org.example.service.model.User;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.ArrayList;
import java.util.List;
@RestController
@RequestMapping("users")
public class UserController {
Logger log = LoggerFactory.getLogger(UserController.class);
@Autowired
private RedisCommands<String,String> commands;
@GetMapping("/data/{username}")
public User getUserData(@PathVariable String username) {
log.info("Get data for username: {}", username);
final User response = new User();
response.setUsername(username);
List<String> privileges = commands.lrange(username, 0L, 1000L);
response.setRoles(privileges);
return response;
}
@PostMapping("/data")
private User createNewUser(String username, String firstRole, String secondRole) {
log.info("Create a new user with the username: " + username + "and roles: " + firstRole + ", " + secondRole);
List<String> roles = new ArrayList<>();
roles.add(firstRole);
roles.add(secondRole);
User user = new User();
user.setUsername(username);
user.setRoles(roles);
commands.lpush(username, firstRole, secondRole);
return user;
}
} -
Last but not least, append the configuration for Redis to the
codenow/config/application.yaml
file.- Note that this configuration depends on your local development setup for Redis and can differ on a case-by-case basis.
- Make sure you follow the yaml syntax (especially whitespaces).
spring:
redis:
url: redis-sentinel://localhost:26379/0#mymaster
sentinel:
master: mymaster
nodes: localhost:26379 -
Try to build and run the application in your IDE. After startup, you should be able to access your new controller’s swagger: http://localhost:8080/swagger/index.html
- For the correct setup, check the
README.md
in the project root or see the tutorial Java Spring Boot Local Development, section Prepare local development IDE - The image below is similar to what your Swagger UI should look like.
- For the correct setup, check the
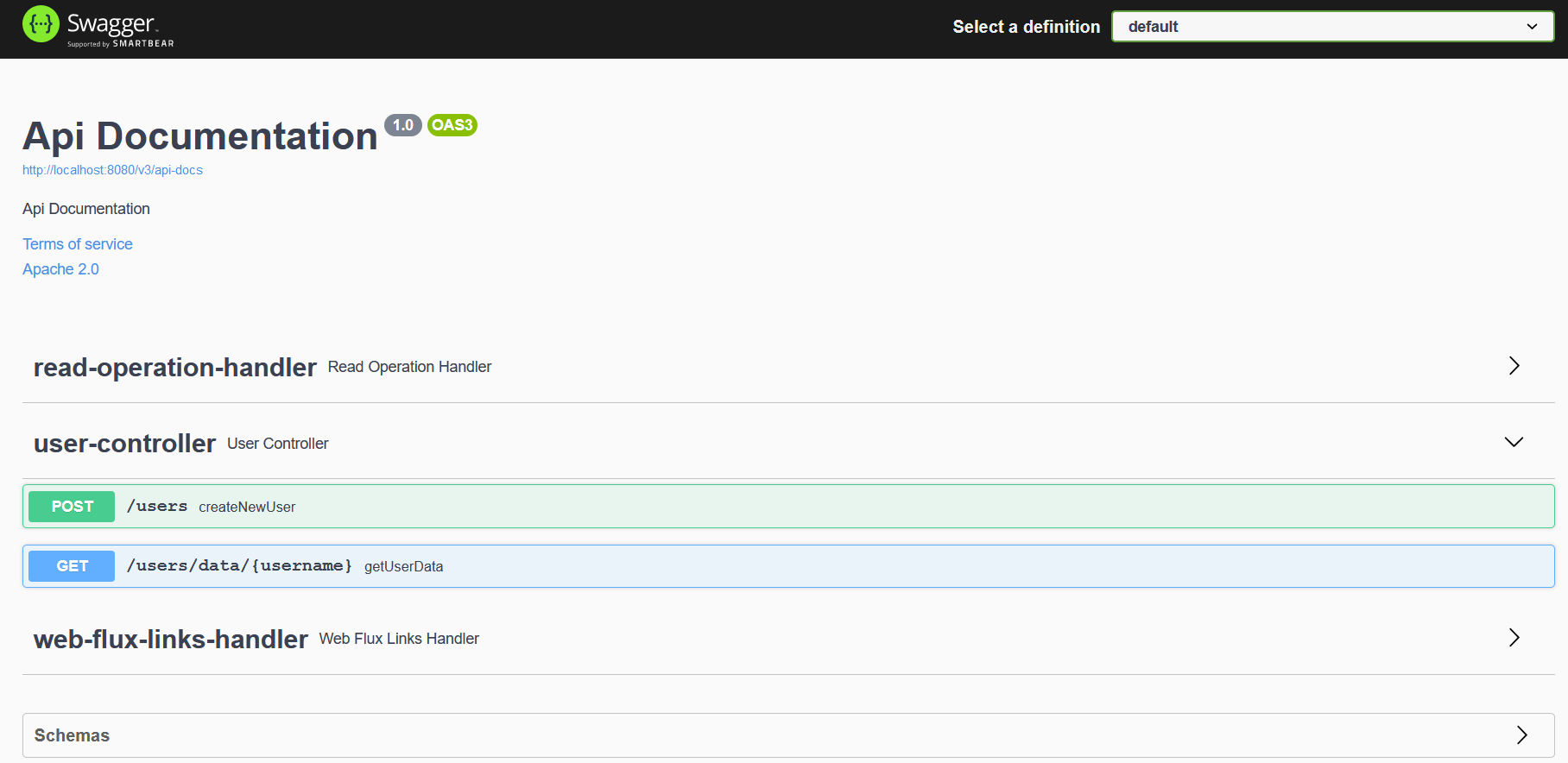
Deploy to CodeNOW​
If your code works in the local development environment, you are ready to push your changes to GIT and try to build and deploy your new component version to the CodeNOW environment.
- Check Get New Redis for setup in the CodeNOW environment.
- Make sure to change the application.yaml properties from the local to the production setup.
- For more information about application deployment, see the Application Deployment and Deployment Monitoring tutorials.
What’s next?​
See our other developer tutorials: