Java Spring Boot REST Server with CockroachDB
đź•“ 45 minutes
What you’ll learn​
How to set up your application for :
- connecting to the CockroachDB database
- getting data from REST API
- providing data to REST API from the database.
In this tutorial, we will create a simple java component with Java Spring Boot scaffolder with a connection to CockroachDB database storage. We want to expose a single REST endpoint for getting the basic client data information, creating a microservice CRUD layer above the DB storage.
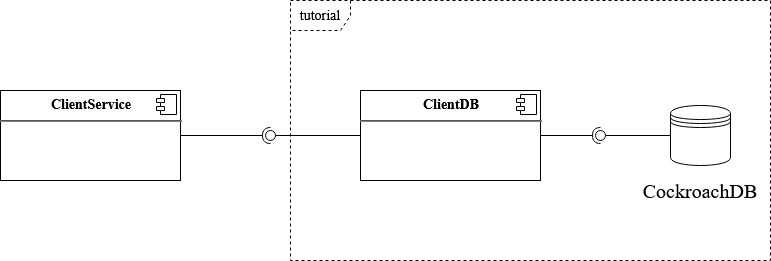
Project source​
This example project can be cloned from: http://gitlab.cloud.codenow.com/public-docs/java-spring-boot-demo/java-spring-boot-rest-server-with-cockroachdb.git
Prerequisites​
- Prepare your local development environment for CodeNOW with Spring Boot.
- Follow the tutorial instructions in the Java Spring Boot Local Development tutorial.
- Run CockroachDB locally.
- You can run CockroachDB directly or using docker compose.
- Instructions on how to use CockroachDB with docker can be found here: https://kb.objectrocket.com/cockroachdb/docker-compose-and-cockroachdb-1151
- Create a new component
- For details, see the section Prerequisites of the Java Spring Boot Local Development tutorial.
Steps​
Open your IDE, import the created component and start coding:
-
Add these maven dependencies to your
pom.xml
file:<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.3.3.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
<version>2.3.3.RELEASE</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.4.21.Final</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.2.11</version>
</dependency> -
Define jpa entity
Client
. This simple table will store the basic client data:- Generate getters and setters with your IDE
- NOTE: if your table name is different from the model class name, you should specify the table name inside the
@Table
annotation.
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "client_data")
public class Client {
@Id @GeneratedValue
private Long id;
private String username;
private String firstname;
private String surname;
//...getters + setters
} -
Create a new
ClientRepository
, which is a basic CRUD interface for Spring Boot data DB access:- For more details about Spring Boot @Repository annotation, see: https://docs.spring.io/spring-framework/docs/current/javadoc-api/org/springframework/stereotype/Repository.html
import org.example.service.model.Client;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface ClientRepository extends JpaRepository<Client, Long> {
Client getClientByUsername(String username);
} -
Create a new controller and put all the parts together
- For more details about Spring Boot controllers, see: https://docs.spring.io/spring/docs/3.0.0.M4/reference/html/ch15s03.html
import org.example.service.model.Client;
import org.example.service.repository.ClientRepository;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController("/clients")
public class ClientController {
private static final Logger LOG = LoggerFactory.getLogger(ClientController.class);
@Autowired
private ClientRepository clientRepository;
public ClientController(ClientRepository clientRepository) {
super();
this.clientRepository = clientRepository;
}
@GetMapping
private ResponseEntity<List<Client>> getAll() {
List<Client> clients = clientRepository.findAll();
if(clients.size() <= 0) {
return new ResponseEntity<>(null, HttpStatus.NO_CONTENT);
}
return new ResponseEntity<>(clients, HttpStatus.OK);
}
@GetMapping("/{username}")
private ResponseEntity<Client> getClientByUsername(@PathVariable String username) {
LOG.info("Get data for username: {}", username);
Client client = clientRepository.getClientByUsername(username);
if(client == null) {
return new ResponseEntity<>(null, HttpStatus.NOT_FOUND);
}
return new ResponseEntity<>(client, HttpStatus.OK);
}
} -
Next prepare the database configuration:
- If you are using docker-compose or regular docker, then go to the cockroach sql terminal and create a new database.
- You can use this link for help: https://kb.objectrocket.com/cockroachdb/docker-compose-and-cockroachdb-1151
- Then create a new table with the name "client" inside your new database and fill it with values.
- If you are using docker-compose or regular docker, then go to the cockroach sql terminal and create a new database.
-
Now change the local configuration in
codenow/config/application.yaml
:-
Fill
{db user}
and{db password}
according to your local development setupdangerThese settings are valid only in your local development environment to connect to your local database! Never store your credentials in the codebase! You don't have to implement your own solution for the secure storage of credentials. In CodeNOW, credentials are securely stored for each deployed service (database/message broker/...). You can find how to set up the service connection in CodeNOW in the Deployment Tutorial and in the Connecting Services Tutorial.
-
Make sure you follow yaml syntax (especially whitespaces)
server:
port: 8080
spring:
main:
banner-mode: off
zipkin:
enabled: false
datasource:
url: jdbc:postgresql://localhost:26257/{db name}}
username: {db user}
password: {db password}
driver-class-name: org.postgresql.Driver
jpa:
properties:
hibernate:
dialect: org.hibernate.dialect.PostgreSQL95Dialect
management:
endpoints:
web:
exposure:
include: health, prometheus -
-
Try to build and run the application in your IDE. After startup, you should be able to access your new controller’s swagger: http://localhost:8080/swagger/index.html
- For correct setup, check the
README.md
in the project root or see the tutorial Java Spring Boot Local Development, section Prepare local development IDE
- For correct setup, check the
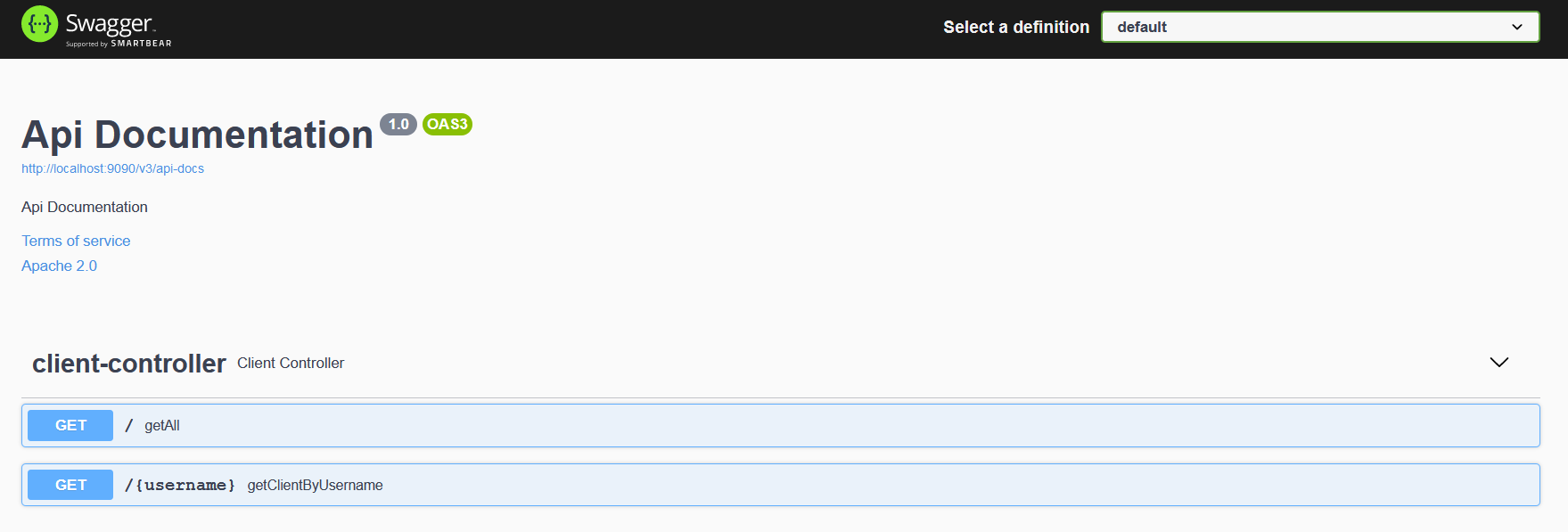
Deploy to CodeNOW​
If your code works in the local development, you are ready to push your changes to GIT and try to build and deploy your new component version to the CodeNOW environment.
- Check the Get New CockroachDB user manual to get CodeNOW managed component properties.
- Make sure to change the application.yaml properties from the local to the production setup.
- For more information about application deployment, see the Application Deployment and Deployment Monitoring tutorials.
What’s next?​
See our other developer tutorials: